Create GitHub issue hierarchy using the API
The newly introduced GitHub Issues updates have added support for issue types as well as parent-child hierarchies. Unfortunately, the GitHub CLI hasn't yet been updated to support setting up this tree structure.
I tried using a direct REST API call, but unfortunately, I haven't been able to get that to work, even though it's documented, whatever I throw at the API, it returns 404:
> "{""sub_issue_id"": 12 }" | gh api https://api.github.com/repos/xebia/temp/issues/165/sub_issues -X post --input -
{
"message": "The provided sub-issue does not exist",
"documentation_url": "https://docs.github.com/rest/issues/sub-issues#add-sub-issue",
"status": "404"
}
gh: The provided sub-issue does not exist (HTTP 404)
Some more searching, brought me to the post introducing the feature, which lists a number of GraphQL API calls you can make.
These API calls don't use the issue number, but instead rely on the issue ID. To get the ID, you'll first need to query the issue details, and you need to pass in an additional preview header to enable the sub_issues
feature in GraphQL:
function create-hierarchy
{
param(
# https://github.com/{org}/{repo}/issues/{id}
[string]$parent,
# https://github.com/{org}/{repo}/issues/{id}
[string]$child
)
$ErrorActionPreference = "Stop"
$parent = gh issue view $parent --json id --jq ".id"
$child = gh issue view $child --json id --jq ".id"
$_ = gh api graphql -H "GraphQL-Features: sub_issues" -f "query=mutation addSubIssue {
addSubIssue(input: { issueId: ""$parent"", subIssueId: ""$child"" }) {
issue {
title
}
subIssue {
title
}
}
}"
}
You can call this in the following manner:
create-hierarchy `
-parent https://github.com/jessehouwing/sample/1 `
-child https://github.com/jessehouwing/sample/2
And you'll end up with this nice list of sub-issues:
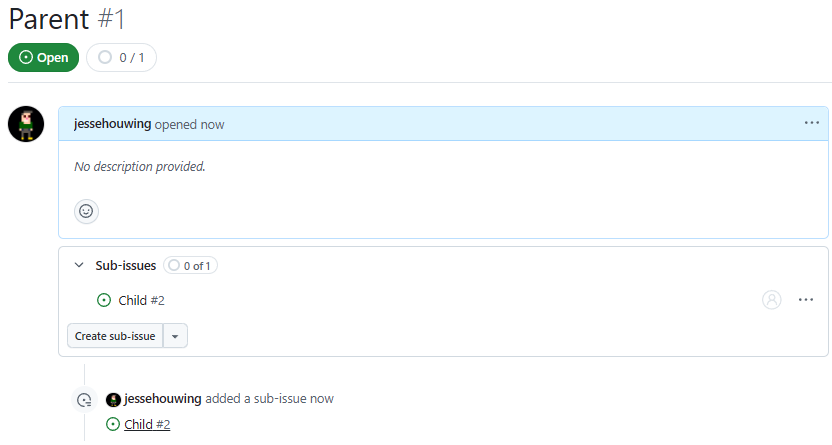
You can nest issues as well; they'll be visible on each level of the hierarchy:
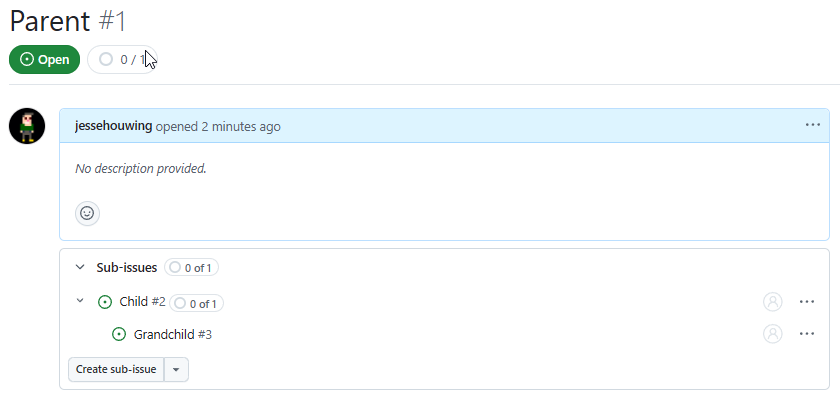
And you can visualize progress in GitHub Projects as well by adding the Parent and Sub issues progress fields to the selected fields:
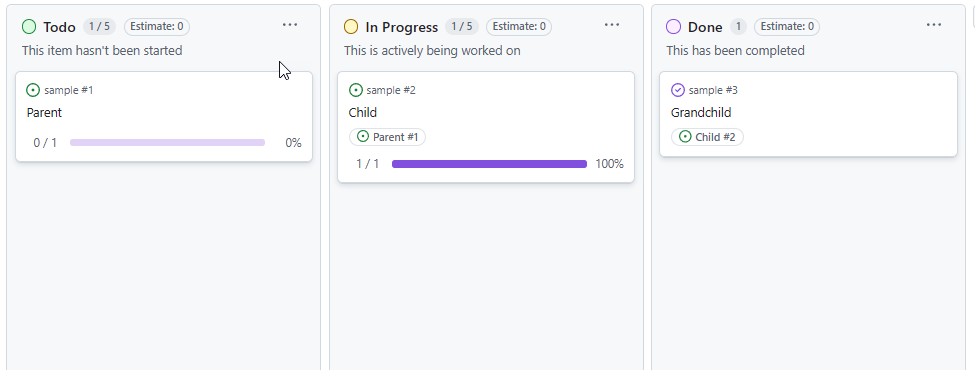
Update
Some more digging lead me to the correct information, it turns out that gh issue view --json id
doesn't return the correct value to pass as sub_issue_id
, if I do a raw REST API all for the issue instead, I receive a different set of numbers where the node_id
is what gh issue view
actually returns:
{
"url": "https://api.github.com/repos/jessehouwing/sample/issues/1",
"id": 3000028010,
"node_id": "I_kwDOOakzpM6yyU6H",
"number": 1
...
}
So to use the REST API call, instead of the GraphQL call, you'll still need to query the id
of the child issue, but then you should be able to directly assign the value:
> gh api https://api.github.com/repos/jessehouwing/sample/issues/1 --jq .id
3000028010
Followed by:
> gh api https://api.github.com/repos/jessehouwing/sample/issues/1/sub_issues -X post -F sub_issue_id=3000028010
Note that the URL contains the issue's number
and the sub_issue_id
is the issue's internal id
.